
First install the package via composer:
$ composer require spatie/laravel-sitemap
- Configuration
If you would like to change the default options, you could publish the config by using the following command:
php artisan vendor:publish --provider="Spatie\Sitemap\SitemapServiceProvider" --tag=config
This will copy the config to config/sitemap.php
where you would be able to change it depending on your needs.
- Usage
To generate a sitemap for your website with all of the found links, you can use the following:
SitemapGenerator::create('https://example.com')->writeToFile(public/sitemap.xml);
- Automation
To automate the process what you could do is create an artisan command and then add it to your scheduler.
To do that just run the following:
php artisan make:command GenerateSitemap
And then update the content of the app/Console/Commands/GenerateSitemap.php
file accordingly. I would usually use something like this:
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Spatie\Sitemap\SitemapGenerator;
class GenerateSitemap extends Command
{
/**
* The console command name.
*
* @var string
*/
protected $signature = 'sitemap:generate';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Generate the sitemap.';
/**
* Execute the console command.
*
* @return mixed
*/
public function handle()
{
// modify this to your own needs
SitemapGenerator::create(config('app.url'))
->writeToFile(public_path('sitemap.xml'));
}
}
Then to generate your sitemap just run:
php artisan sitemap:generate
To schedule this to run on daily basis just add the following to your app/Console/Kernel.php
file:
protected function schedule(Schedule $schedule)
{
...
$schedule->command('sitemap:generate')->daily();
...
}
That is pretty much it, then your sitemap.xml should be available at example.xml/sitemap.xml
.
For more information I would recommend going through the official repo here:
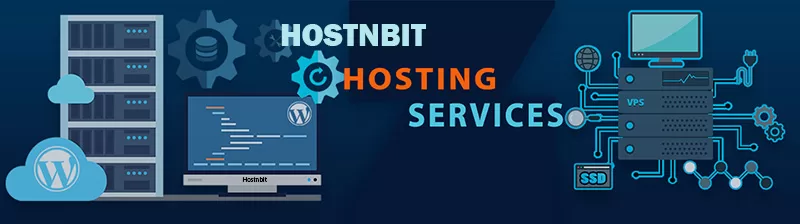