
We recently created a new website for Ashton & Partners, a design firm located in downtown San Francisco. The Ashton & Partners home page features a series of images that slowly cross-fade from one to the next, all of which can be controlled via a custom WordPress control panel. In designing the site, it was important that the site work on devices that do not support Flash (i.e. – iPhone, iPad, etc.) so we chose to create all site animations using JavaScript.
While there are thousands of pre-built animation scripts out there, most of the scripts I found were overblown or not exactly what we needed. Below are the component parts for our custom infinite loop cross-fade script (HTML, CSS, and Javascript) plus links to a working demo and source files. Note: by applying the “rotating-item” class, the script can be used to infinitely loop over any set of HTML elements (paragraphs, divs, etc.), we just happen to target images in this example.
The HTML
We start with a basic HTML5 doctype. In the head of the document, we link to the jQuery JavaScript library via Google AJAX APIs and include our ‘infinite-rotator.js’ file. Note: for slightly better page load speed you can link to the Javascript files at the bottom of the HTML instead. We then create a list of images and assign each a class of “rotating-item”. We’ll use this class to control the images via CSS and JavaScript later. Finally, we wrap all of our rotating images in a DIV we give an id of “rotating-item-wrapper” which we explain in the CSS section. Unstyled, the HTML will display the entire series of images (view unstyled page).
Infinite Rotating Images Using jQuery (JavaScript) <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js?ver=3.0.1"></script><script type="text/javascript" src="js/infinite-rotator.js"></script> </pre> <h1>Infinite Rotating Images Using jQuery (JavaScript)</h1> <div id="rotating-item-wrapper"><img class="rotating-item" src="images/greenpeople.jpg" alt="a person entering a building" width="980" height="347" /> <img class="rotating-item" src="images/entrance.jpg" alt="photo of building across from our office" width="980" height="347" /> <img class="rotating-item" src="images/bluepeople.jpg" alt="building entrance with neon backlit walls" width="980" height="347" /> <img class="rotating-item" src="images/reflection3.jpg" alt="building lobby window reflections" width="980" height="347" /> <img class="rotating-item" src="images/reflection2.jpg" alt="reflection on building windows" width="980" height="347" /> <img class="rotating-item" src="images/manequine.jpg" alt="two manequines in window" width="980" height="347" /></div> <pre>
The CSS
Our CSS code is simple and basically does two things — it absolutely positions all of our rotating items on top of each other in the wrapper DIV and hides the items using CSS display=none.
#rotating-item-wrapper { position: relative; width: 980px; height: 347px; } .rotating-item { display: none; position: absolute; top: 0; left: 0; }
The JavaScript
This is the heart of the rotating image functionality. Now that we have our basic HTML markup and have absolutely positioned and hidden our images using CSS, we can now rotate between them. We start our JavaScript only when the HTML document and images have finished loading by using the window load function. For those familiar with jQuery, this is a bit different than the document ready command which fires after the document is ready, not when linked images have loaded which is important here. (Note: the same can be achieved by linking the JavaScript files at the bottom of the HTML versus in the head of the document.) We then define a few timing variables — the initial fade-in time, the interval between items, and the cross-fade time — all set in milliseconds. Adjust as desired. On line 23, we reveal the first image, then on line 26 kick off our infinite loop.
$(window).load(function() { //start after HTML, images have loaded var InfiniteRotator = { init: function() { //initial fade-in time (in milliseconds) var initialFadeIn = 1000; //interval between items (in milliseconds) var itemInterval = 5000; //cross-fade time (in milliseconds) var fadeTime = 2500; //count number of items var numberOfItems = $('.rotating-item').length; //set current item var currentItem = 0; //show first item $('.rotating-item').eq(currentItem).fadeIn(initialFadeIn); //loop through the items var infiniteLoop = setInterval(function(){ $('.rotating-item').eq(currentItem).fadeOut(fadeTime); if(currentItem == numberOfItems -1){ currentItem = 0; }else{ currentItem++; } $('.rotating-item').eq(currentItem).fadeIn(fadeTime); }, itemInterval); } }; InfiniteRotator.init(); });
That’s it! I hope you found this example useful.
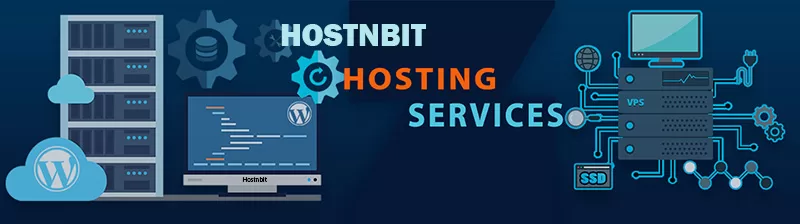